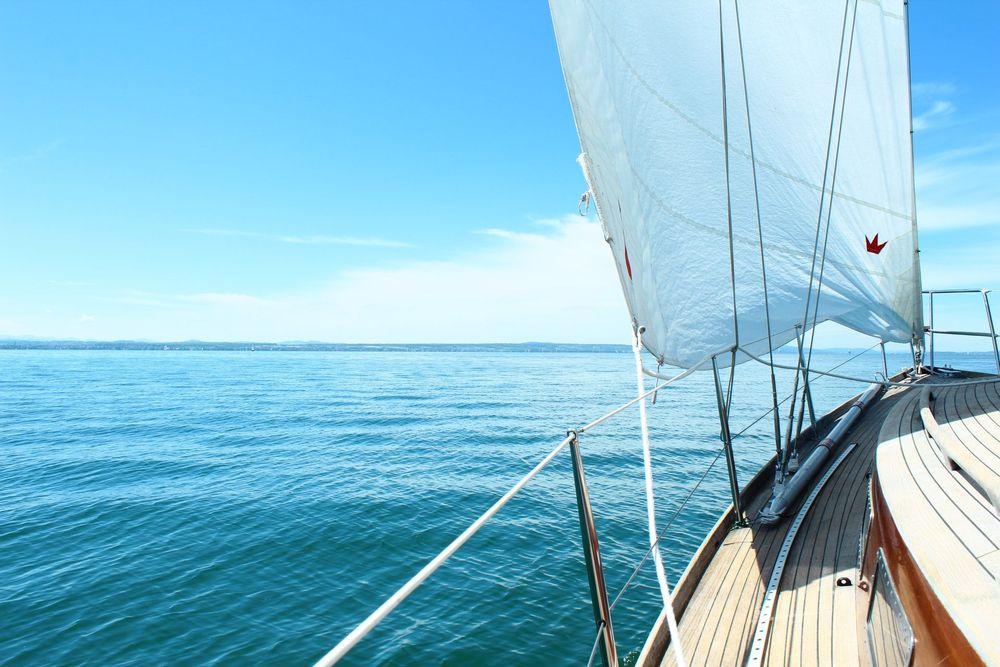
Configuring MDX for Next.js
MDX allows you to write JSX in Markdown documents. You can embed these components in your document. In this article, I show how to configure your Next.js application to work with MDX.
Installing
For the MDX integration, you need to install the following packages:
npm install --save @next/mdx @mdx-js/loader next-mdx-remote
Note that next-mdx-remote is published under Mozilla Public License (MPL).
Configuration of .mdx as Pages
Next, you need to configure the .mdx files in the next.config.js file:
next.config.js
const withMDX = require('@next/mdx')({
extension: /\.mdx$/
})
module.exports = withMDX({
pageExtensions: ['js', 'jsx', 'mdx']
})
Rendering MDX
Then, configure your page component to use and render the contents of the MDX file:
Taken from the next-mdx-remote example:
import { serialize } from 'next-mdx-remote/serialize'
import { MDXRemote } from 'next-mdx-remote'
import Test from '../components/test'
const components = { Test }
export default function TestPage({ source }) {
return (
<div className="wrapper">
<MDXRemote {...source} components={components} />
</div>
)
}
export async function getStaticProps() {
// MDX text - can be from a local file, database, anywhere
const source = 'Some **mdx** text, with a component <Test />'
const mdxSource = await serialize(source)
return { props: { source: mdxSource } }
}
Instead of using a static text in the getStaticProps() like in this example, you can read from the MDX text files and serialize the content.
Conclusion
MDX is very useful when you want to turbocharge your Markdown documents. With next-mdx-remote it is possible to easily use MDX in your Next.js application.
References
-
Markdown: https://www.markdownguide.org/
-
MDX: https://mdxjs.com/
-
next-mdx-remote: https://github.com/hashicorp/next-mdx-remote
Published
28 Jul 2021