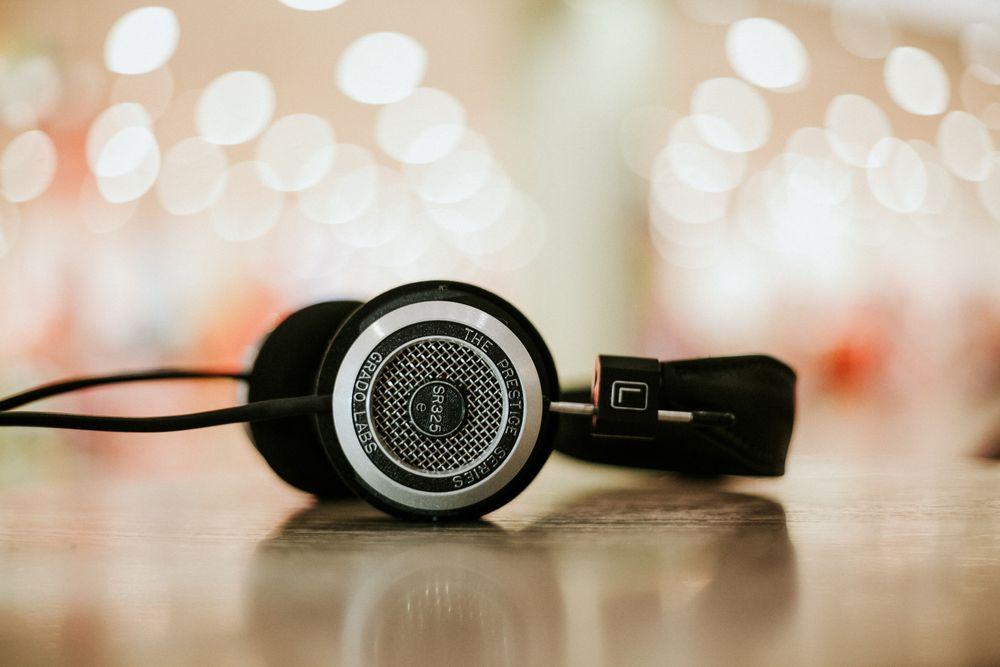
How to Receive Audio Messages from Your Users
Everybody knows the contact forms on websites where users can send text message to the website owner. But how could you design a contact form that allows the recording of an audio message? In this article, I want to show you how to do that.
What is Audio Tapir
Audio Tapir is a project that enables developers to quickly create a system that allows a website user to send a contact audio message to the website owner. The tapir is a cute animal from the South American jungle and is thought to eat people's nightmares.
It consists of a frontend part written in Vue 3 and a serverless backend function. The frontend part is called vue-audio-tapir and can easily be integrated into your website. The backend part is a serverless Netlify function called audio-tapir-function.
The frontend part communicates with a POST call with the serverless function and transmits the recorded audio data. In the Netlify function this audio data is packaged as an email attachment and sent to the recipient, normally the owner of the website.
You can see a picture of the general architecture as follows:
Vue-audio-tapir
The frontend component written in Vue 3 (vue-audio-tapir) uses the Web Audio API to ask for permission to use the microphone. Subsequently, the user can record the message. The message is encoded as MP3 data using the lamejs library for more efficient storage. The included audio player allows the user to play back his recorded message. When everything is correct, he can submit the audio data, and it is sent to the backend server for further processing.
You can integrate the vue-audio-tapir like this into your Vue 3 application:
<template>
<tapir-widget :time="2" backendEndpoint="https://your-endpoint.com/.netlify/functions/audio-message" />
</template>
<script>
import TapirWidget from 'vue-audio-tapir';
import 'vue-audio-tapir/dist/vue-audio-tapir.css';
export default {
name: 'App',
components: {
TapirWidget,
}
}
</script>
Before that, you of course need to install vue-audio-tapir using npm or yarn, like so:
npm i vue-audio-tapir --save
The most important property of the component is the backendEndpoint which is the URL of your backend server that does the audio data processing.
Other properties are documented on the GitHub page of vue-audio-tapir.
Audio-tapir-function
The backend function is called audio-tapir-function and is based on Netlify. Prior to deploying the function on a server, you need to register at Netlify's website. They have a free tier of serverless functions.
The function uses SendGrid to send the audio message to a recipient. For easy playback, the message is packaged as an attachment in the email. Three environment variables need to be present and must be added in the Netlify dashboard for the deployed function.
import { Handler } from "@netlify/functions";
import sendgridMail from "@sendgrid/mail";
sendgridMail.setApiKey(process.env.SENDGRID_API_KEY);
export const handler: Handler = async (event) => {
const audioBody = event.body;
const msg = {
to: process.env.EMAIL_TO,
from: process.env.EMAIL_FROM,
subject: "Audio Contact Message from Website",
text: `Audio Message: `,
html: `Audio Message: `,
attachments: [
{
content: audioBody,
filename: "audio.mp3",
type: "audio/mpeg",
disposition: "attachment"
}
]
};
try {
await sendgridMail.send(msg);
} catch (error) {
console.error(error);
if (error.response) {
console.error(error.response.body);
return {
statusCode: 400,
headers: {
'Access-Control-Allow-Origin': '*', // Required for CORS support to work
'Access-Control-Allow-Headers': '*',
},
body: JSON.stringify({
message: 'Error: '+JSON.stringify(error.response.body)
}),
};
}
}
return {
statusCode: 200,
headers: {
'Access-Control-Allow-Origin': '*', // Required for CORS support to work
'Access-Control-Allow-Headers': '*',
},
body: JSON.stringify({
message: 'Success!'
}),
};
};
You can try out the function locally on your machine by installing Netlify's CLI tool. With this tool, you can also quickly deploy the function to production.
Conclusion
With this project, I hope I could show you that the integration of the useful functionality of audio contact messaging in your websites is straightforward. I am sure that this possibility adds value for your customers and will distinguish your customer service from other websites.
References
-
vue-audio-tapir: https://github.com/tderflinger/vue-audio-tapir
-
audio-tapir-function: https://github.com/tderflinger/audio-tapir-function
-
Vue 3: https://v3.vuejs.org/
-
lamejs: https://github.com/zhuker/lamejs
-
Netlify Functions: https://functions.netlify.com/
-
SendGrid: https://sendgrid.com/
-
Photo of tapir by Get_It with CC-SA license
Photo of Alphacolor on Unsplash
Published
28 Nov 2021