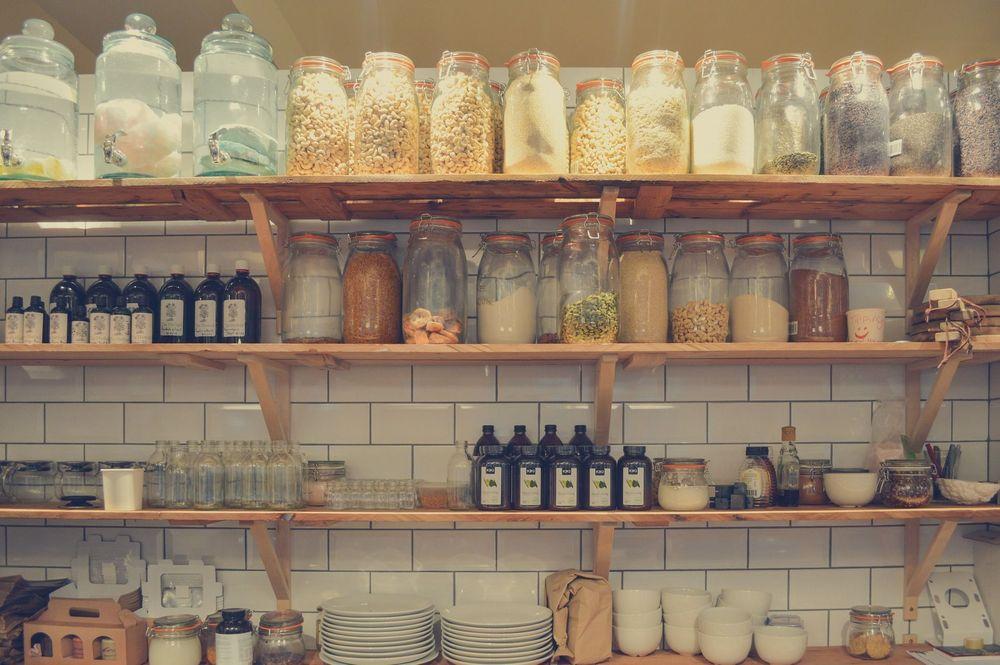
Gatsby with Google Cloud Storage and Terraform
With Gatsby, you can generate a static website that works great with cloud storage solutions like Google Cloud Storage. How to configure Gatsby and use Infrastructure as Code tools like Terraform I want to show in this article.
Google Cloud Storage
In Google Cloud Storage (GCS) you can store files of any type (binary and text) on Google's servers. The storage is organized in buckets, which are referenced by globally unique identifiers. Within each bucket, you can have multiple files and directories. There are different types of buckets, depending on use case. For example, you can have single region or multiple region replication, buckets for archival purposes and other needs.
You can manage the buckets via a web interface or using the REST API and a command line application (gsutil).
The following picture is taken from the web interface storage browser where you can manage your buckets.
For the creation of the example bucket, we will use a popular tool called Terraform.
Terraform
Manually configuring cloud services is fine for getting to know them. But when you work with cloud services on a daily basis, you rather need to find a way to automate your work. This is the mantra of Infrastructure as Code (IaC). That means you programmatically create the infrastructure and thus can easily automate tasks. This saves time and increases reliability. One tool to enable IaC is Terraform.
Behind the scenes, Terraform invokes the REST APIs of the respective services. That means in most cases you need to set up the environmental variables for the API keys and install the CLI tools. For GCS, you can follow this tutorial: https://cloud.google.com/sdk/
You also need to install the Terraform application (https://learn.hashicorp.com/terraform/getting-started/install), which is just one file.
Since we want to deploy a static Gatsby website, all files need to be publicly visible. Thus, there is a public_rule resource defined that makes the web files publicly readable.
This is the Terraform definition file:
google-bucket.tf
resource "google_storage_bucket_access_control" "public_rule" {
bucket = google_storage_bucket.static-site.name
role = "READER"
entity = "allUsers"
}
resource "google_storage_bucket" "static-site" {
name = "test-yoursite-com"
project = "yoursite"
location = "EU"
force_destroy = true
storage_class = "STANDARD"
website {
main_page_suffix = "index.html"
not_found_page = "404.html"
}
}
Replace test-yoursite-com with the unique ID of your own Gatsby site.
After you run terraform init and terraform apply, the bucket test-yoursite-com will be created on GCS.
You can confirm the successful creation by opening the storage browser in the Google Cloud Console:
https://console.cloud.google.com/storage
Gatsby
Gatsby is a static website generator. For these kinds of websites, no real server is needed. Instead, cloud storage solutions like Google Cloud Storage are ideal. The amount of money you pay compared to running a full server is minimal.
One thing to note when using Gatsby with Google Cloud Storage is that by default, all websites are hosted by their bucket name under the https://storage.googleapis.com/ domain.
For example, the test bucket would be hosted under: https://storage.googleapis.com/test-yoursite-com
For this to work in Gatsby, you need to add one option in the gatsby-config.js:
gatsby-config.js
module.exports = {
pathPrefix: "/test-yoursite-com",
...
Then you need to generate your Gatsby site with
gatsby build --prefix-paths
That ensures that the file paths and links are created correctly.
After that, simply upload the contents of your Gatsby public folder to the bucket with the Google Cloud CLI command:
cd public
gsutil cp -r . gs://test-yoursite-com
gsutil iam -r ch allUsers:objectViewer gs://test-yoursite-com
The second gsutil command makes all files publicly viewable.
Conclusion
With Gatsby, you can create static sites that work well with cloud storage solutions like Google Cloud Storage. Using Terraform automates the creation of buckets and makes it reproducible.
References
-
Google Cloud Storage: https://cloud.google.com/storage/
-
Terraform: https://www.terraform.io/
-
Gatsby: https://www.gatsbyjs.org/
Published
28 Mar 2020