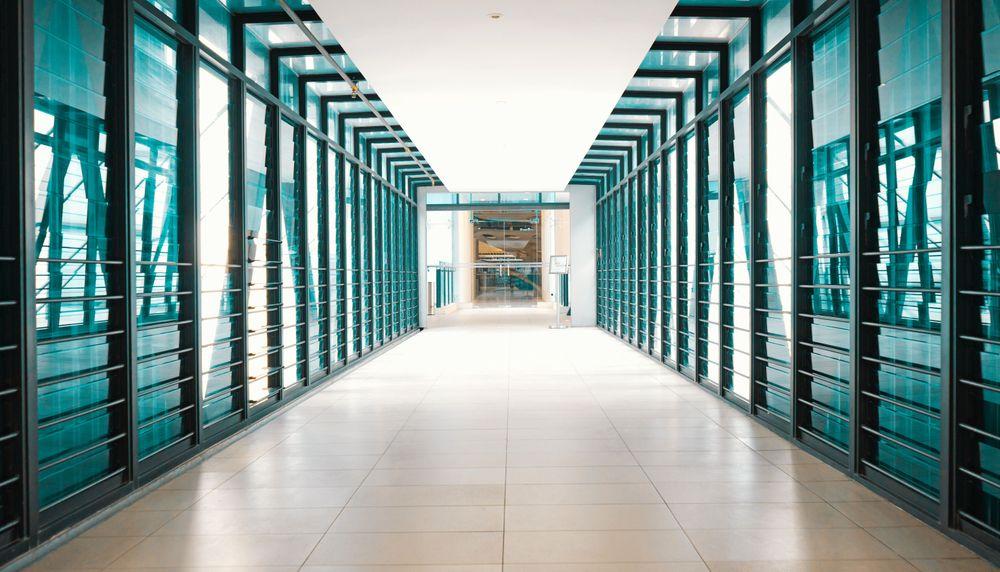
Serverless for Contact Messaging
Serverless is a framework for building and deploying serverless code. Provisioning and scaling is automatically handled by the cloud provider. The Serverless framework is an abstraction on top of the most important cloud providers. This tutorial will show how to write a function that sends an email with customer contact information to you. This is a popular use case for contact forms on websites.
For the purpose of this tutorial, the Twilio Sendgrid API will be used to send an email. This tutorial requires some basic knowledge of JavaScript and API calls.
Serverless Computing
Serverless computing, also known as function as a service (FaaS) was first pioneered by Amazon AWS with AWS Lambda in 2014. Despite its name serverless, there are servers involved. But the developer does not need to care for this server. She can write the server code and then run a command to automatically deploy the function. Scaling is handled by the cloud provider. Often, the pricing is based on function call number and memory or CPU time.
This means fixed server costs that traditionally were calculated based on how long the server runs are now transformed in variable costs based on the number of calls and memory or CPU.
The new use cases for serverless computing are for example resizing an image after upload or sending an email to the service department when the customer enters his contact information and message on a website contact form.
This tutorial describes the latter use case. Other use cases include event streaming, and more are listed on the Serverless website.
Serverless Framework
The Serverless framework is a free and open source framework written in Node.js. It supports all major cloud providers like AWS, Google Cloud, Azure, Cloudflare Workers and Alibaba Cloud. There is also an enterprise version that the company behind the Serverless framework, Serverless Inc. supports. Serverless Inc. is a startup based in San Francisco, USA and has the backing of Trinity Ventures, among others.
The installation of Serverless is pretty straightforward. Just follow the instructions of the Serverless docs page.
Next, clone the serverless-message example application from the GitHub repository. Run npm install and this resolves the package dependencies.
Configuration
All major configuration is done in the file serverless.yml. There, you can find information on which cloud provider to use, which runtime and which region. Under functions the name of the function is specified, its http method (POST) and that CORS should be enabled. Also, this is a private function, meaning the caller needs to include the API key in the header of the POST call. The value of the API key is in the apiKeys value section.
serverless.yml
service: serverless-message
provider:
name: aws
runtime: nodejs8.10
region: eu-central-1
apiKeys:
- name: firstKey
value: JNJSNKMKDMKSMKMDKMDK
environment:
SENDGRID_API_KEY: Your API Key
MESSAGE_TO: Your email address
MESSAGE_FROM: The sender email address (your website domain)
usagePlan:
quota:
limit: 5000
offset: 2
period: MONTH
throttle:
burstLimit: 20
rateLimit: 10
functions:
sendMessage:
handler: handler.sendMessage
events:
- http:
path: send-message
method: post
cors: true
private: true
plugins:
- serverless-offline
There are also three variables defined that need to be set by you.
The SENDGRID_API_KEY
is your Sendgrid API key. It can be created after signing
up to Twilio Sendgrid.
The other two environment variables are the email address where the
contact message of the client should be sent to. This is normally
your business email address. There you will receive the message when
a client submits on your website. The MESSAGE_FROM
variable
is the email address of your website.
The other section is the usagePlan that is a security feature where you can set the maximum number of monthly invocations.
Serverless Function
This is the meat of the application. The handler function is just a normal JavaScript function. You can import code from Node packages. In this case, the @sendgrid/mail is needed for sending out the email.
Otherwise, the function is simple. It sends an email with the data (name, email, message) that was included as a body of the POST call.
The frontend will later need to post the data from the information that the customer entered in a form. Currently, the frontend is not part of this application.
handler.js
'use strict'
const sgMail = require('@sendgrid/mail')
function sendEmail(data) {
sgMail.setApiKey(process.env.SENDGRID_API_KEY)
const msg = {
to: process.env.MESSAGE_TO,
from: process.env.MESSAGE_FROM,
subject: 'New Message from Website',
text: `Message from ${data.name} - ${data.email}: ${data.message}`,
html: `<strong>Message from ${data.name} - ${data.email}: ${data.message}</strong>`,
}
sgMail.send(msg)
}
module.exports.sendMessage = (event, context, callback) => {
const data = JSON.parse(event.body)
sendEmail(data)
const response = {
statusCode: 201,
headers: {
'Access-Control-Allow-Origin': '*', // Required for CORS support to work
},
body: JSON.stringify({
message: 'Message sent successfully.',
input: event,
}),
}
callback(null, response)
}
The function return a 201 success code with a success message.
Testing Offline
The good thing about Serverless is that it has a good plugin ecosystem. There is also a very useful plugin for testing the AWS serverless function locally on your PC. This plugin is called serverless-offline.
You can run the function locally with:
sls offline
This starts a local server and also prints the API key. You can for example use Postman to run a POST command against the server. You need to include the API key in the header (x-api-key).
Here is an example POST message body:
{
"email": "[email protected]",
"name": "Superman",
"message": "Greetings to Nietzsche!"
}
When you do that, an email with this information will be sent to your configured email address.
Deploying
Deploying is easy but can take a bit of time since all resources need to be uploaded and provisioned.
Just run the command:
sls deploy
Please note that you need a functional AWS CLI installed locally, and the AWS user needs to have the correct rights to install AWS lambda functions. Refer to the AWS documentation and the tutorials on Serverless listed below.
Conclusion
Serverless greatly simplifies the development of serverless functions. Also, it enables you to relatively easily switch cloud providers, avoiding vendor lock-in. As an open source framework it has a big ecosystem, is popular and has the backing of a commercial company.
Sources and Further Reading
-
Serverless: https://serverless.com
-
Serverless Offline: https://github.com/dherault/serverless-offline
-
Twilio Sendgrid: https://www.twilio.com/sendgrid
-
Tutorials as introduction to Serverless: https://hackernoon.com/best-tutorials-to-get-started-with-serverless-97c86623cdc0
-
serverless-message example application: https://github.com/tderflinger/serverless-message
Published
12 Nov 2019